[Header
title:"Take a walk on the Idyll side"
subtitle:"Exploring new ways to create interactive documents"
author:"Matthew Conlen"
authorLink:"https://twitter.com/mathisonian" /]
The code blocks with a beige border show the Idyll markup used to create the output you see.
Idyll is an open-source markup language and toolkit for producing interactive web pages. You give Idyll a markup file, and it compiles that file to a full bundle of HTML, JavaScript, and CSS that can run in anyone’s web browser.
The fastest way to get started using Idyll is to install it from npm.
$ npm install -g idyll # install the command line tool
$ echo “# hello world” > index.idl # create an input file
$ idyll index.idl --watch # compile the file and open it in a web browser
We’ve spent too long being forced to choose between a nice authoring environment and a nice programming interface.
Other tools try to address this in different ways. Static blog engines enable people to write clean, concise markup that can be rendered on the web, but often don’t allow for dynamic elements without advanced customization. Wordpress is widely used and highly-customizable, but dynamic content is far removed from the contents of posts, typically shimmed in or inserted via an iframe.
Notebook-style tools like Jupyter are great for analysis, but treat presentation as an afterthought. They don’t do a good job of offering a way to break out of the code-cell metaphor or to run client-side code separate from JavaScript generated during analysis.
Several years ago Wolfram released their Computable Document Format (CDF), meant to “power the next generation of interactive documents, blogs, reports, presentations, articles, books”, along with a proprietary CDF viewer, but failed to acknowledge that a great interactive document format was already available (HTML/JavaScript), along with a very widely distributed viewer (the web browser).
Idyll makes it easier to write documents for the web, building on powerful open-source tools.
## Clean Markup
Idyll starts with the same principles as [markdown](https://daringfireball.net/projects/markdown/syntax), and uses a lot of the same syntax.
If you want text to appear in your output, just start writing text, save the mucking about with `<div>`'s, containers, and `<p>` tags for a different time.
Idyll starts with the same principles as markdown,
and uses a lot of the same syntax. If you want text to appear in your output, just start writing text, save the
mucking about with <div>
’s, containers, and <p>
tags for a different time. Markup should be
written to be read by humans, not just computers.
For a complete listing of markdown syntax that can be used within Idyll documents see the “Repertoire” example, and the markup used to create it.
The real power of Idyll comes when you want to use JavaScript to enrich your writing. Special syntax allows you to embed JavaScript inline with your text. Idyll comes with a variety of components that can be used out-of-the-box to create rich documents.
For example, the `Equation` component can be used to typeset equations
using the [KaTeX library](https://github.com/Khan/KaTeX).
[Equation]
y = \int x^2 dx
[/Equation]
For example, the Equation
component can be used to typeset equations
using the Katex library.
All of the built-in components are documented on Idyll’s website. For a more in-depth discussion of the syntax see:
https://idyll-lang.github.io/syntax.
Under the hood Idyll compiles the static markup into a dynamic React application. This allows writers to easily include any of the thousands of freely available open-source React components in their projects.
For example, react-minimal-pie-chart
is a React component that makes pie charts.
It is unaffiliated with Idyll but included here to show how
third-party components can be used.
To include it on a page, first install it,
$ npm install --save react-minimal-pie-chart
and then include it in your markup. The component is instantiated and displayed on your page:
The component is instantiated and displayed on your page:
[ReactSimplePieChart
slices:`[{
color: '#7b3af5',
value: 0.1,
}, {
color: '#EAE7D6',
value: 0.9, },
]` /]
Idyll can automatically find components installed via npm, so in most cases you won’t need to do any additional configuration after installing — just add the tag to your markup.
Idyll provides special wrappers that help integrate popular JavaScript libraries like D3 and regl. And you can write your own custom components as well.
In addition to helping manage and instantiate components on a page, Idyll makes it easy to manipulate and display variables.
[var name:"x" value:0 /]
For example, I can initialize a variable, and bind its value to a range slider. Whenever the slider is moved, the variable is automatically updated, and anything that depends on that variable is updated as well.
Update x:
0 [Range value:x min:0 max:100 /] 100
Value of x: [DisplayVar var:`x` /].
Update x:
0100
Value of x: 0.00.
Derived variables can be used to
achieve spreadsheet-like functionality. For example, I can define y
as
[derived name:"y" value:`100 - x`/]
Update x:
0 [Range value:x min:0 max:100 /] 100
Value of x: [DisplayVar var:`x` /].
Value of y: [DisplayVar var:`y` /].
Update x:
0100
Value of x: 0.00. Value of y: 100.00.
Variables can be passed into components (try moving the sliders above and watching the pie chart).
[ReactSimplePieChart
slices:`[{
color: '#7b3af5',
value: x,
}, {
color: '#EAE7D6',
value: y, },
]` /]
There are many ways to manipulate variables, and any component has the ability to update a variable. For example, instead of using a range slider, we could define one button that divides the value of x
in half every
time it gets clicked, and a second button that resets the value to 50:
[button onClick:`x = x / 2` ]
Divide!
[/button]
[button onClick:`x = 50` ]
Reset!
[/button]
Value of x: 0.00. Value of y: 100.00.
Idyll provides the ability to load datasets from csv
or json
files.
Once they are loaded, datasets are treated just like variables.
Here I load a dataset about cars and show the first 10 rows in a table:
[data name:"cars" source:"cars.json" /]
[Table data:`cars.slice(0, 10)` /]
Here I load a dataset about cars and show the first 10 rows in a table:
Name | Miles_per_Gallon | Horsepower | Year | Origin |
---|---|---|---|---|
chevrolet chevelle malibu | 18 | 130 | 1970 | USA |
buick skylark 320 | 15 | 165 | 1970 | USA |
plymouth satellite | 18 | 150 | 1970 | USA |
amc rebel sst | 16 | 150 | 1970 | USA |
ford torino | 17 | 140 | 1970 | USA |
ford galaxie 500 | 15 | 198 | 1970 | USA |
chevrolet impala | 14 | 220 | 1970 | USA |
plymouth fury iii | 14 | 215 | 1970 | USA |
pontiac catalina | 14 | 225 | 1970 | USA |
amc ambassador dpl | 15 | 190 | 1970 | USA |
$ npm install --save idyll-vega-lite
I can use Vega-Lite to quickly create a visualization of the data. Idyll has a component specifically for embedding Vega-Lite charts in your documents. It doesn’t ship by default, so first install it, then include it in the markup.
[IdyllVegaLite data:cars spec:`{
width: 300,
height: 200,
renderer: 'svg',
mark: "circle",
encoding: {
x: {field: "Horsepower", type: "quantitative"},
y: {field: "Miles_per_Gallon", type: "quantitative"}
}
}` /]
Vega-Lite makes it easy to iterate on the
design of your visualization by just tweaking a few parameters in the spec
. You can even have it change at runtime. Here I use a variable
to change how the scatterplot is colored:
[var name:"colorBy" value:`{}` /]
[IdyllVegaLite data:cars spec:`{
width: 300,
height: 200,
mark: "circle",
encoding: {
x: {field: "Horsepower", type: "quantitative"},
y: {field: "Miles_per_Gallon", type: "quantitative"},
color: colorBy
}
}` /]
Color by:
[button onClick:`colorBy = {}` ]None[/button]
[button onClick:`colorBy = {field: 'Year', type: 'nominal'}` ]Year[/button]
[button onClick:`colorBy = {field: 'Origin', type: 'nominal'}` ]Origin[/button]
Color by:
I’m excited to see where Idyll goes, and what people do with it. There are lots of possibilities such as making blog engines that can create much richer output, integrating with things like Google Docs, and maybe even supporting React Native.
Check out some more examples of what you can do with Idyll at https://idyll-lang.github.io/. If you use Visual Studio Code, check out the Idyll Syntax plugin to get syntax highlighting for your Idyll documents.
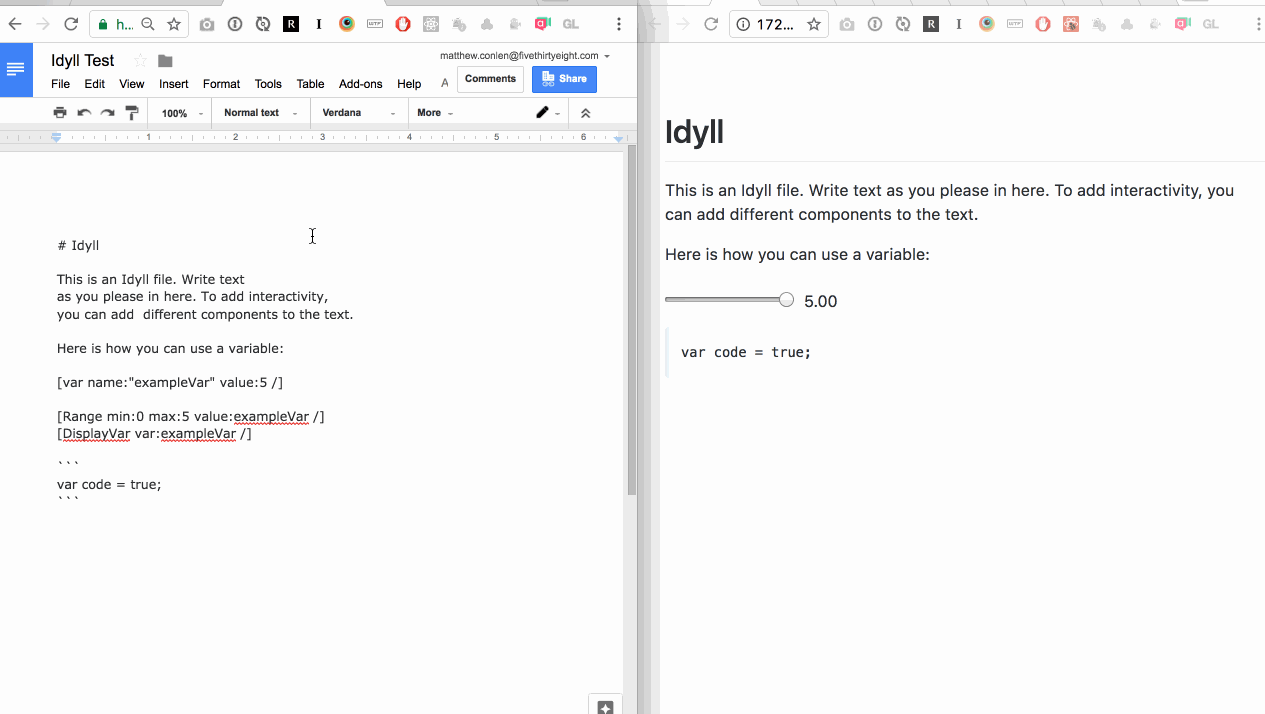
[small]
A clean markup language makes it is possible to use applications like Google Docs as your editor.
[/small]
I’ll be pushing forward on some updates to make it even easier to create documents with a variety of different scroll-based animations, so look out for that in the near future.
If you are interested in the project, please drop by our chatroom to say “Hi!“, and visit us on GitHub.
StarView the full source for this article on GitHub.